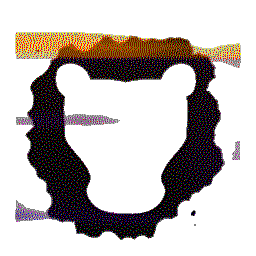 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <boost/property_tree/ptree_fwd.hpp>
25 #include <zmqpp/zmqpp.hpp>
44 const boost::property_tree::ptree &cfg);
75 bool handle_save(zmqpp::message *msg_in, zmqpp::message *msg_out);
104 zmqpp::message *message_out);
112 zmqpp::message *msg_out);
128 std::list<std::string> &remote_modules);
149 std::list<std::string> &stop_list);
173 std::map<std::string, std::function<bool(zmqpp::message *msg_in,
174 zmqpp::message *msg_out)>>;
bool gather_remote_module_list(zmqpp::socket &s, std::list< std::string > &remote_modules)
Build the list of modules (their name) running on the remote host.
zmqpp::context & context_
bool receive_remote_config(zmqpp::socket &s, std::map< std::string, bool > &cfg)
After sending a bunch of MODULE_CONFIG command, wait for reply.
void module_config(const std::string &module, ConfigManager::ConfigFormat cfg_format, zmqpp::message *message_out)
Implements the MODULE_CONFIG command.
void process_config(const boost::property_tree::ptree &cfg)
bool handle_config_version(zmqpp::message *msg_in, zmqpp::message *msg_out)
Command handler for CONFIG_VERSION command.
Provide some kind of security framework to the Remote Control service.
ConfigFormat
This enum is used internally, when core request module configuration.
std::map< std::string, std::function< bool(zmqpp::message *msg_in, zmqpp::message *msg_out)> > CommandHandlerMap
std::string current_client_idt_
void handle_msg()
Register by core and called when message arrives.
bool handle_sync_from(zmqpp::message *msg_in, zmqpp::message *msg_out)
Extract and verify content from message and call sync_from()
bool handle_save(zmqpp::message *msg_in, zmqpp::message *msg_out)
Save the current configuration to disk.
bool handle_general_config(zmqpp::message *msg_in, zmqpp::message *msg_out)
Command handler for GENERAL_CONFIG.
zmqpp::socket socket_
Public ROUTER.
This is the header file for a generated source file, GitSHA1.cpp.
std::string secret_key_
z85 encoded private curve key
RemoteControl(zmqpp::context &ctx, Kernel &kernel, const boost::property_tree::ptree &cfg)
void module_list(zmqpp::message *message_out)
Implements the module list command.
bool gather_remote_config(zmqpp::socket &s, std::list< std::string > &start_list, std::list< std::string > &stop_list)
Will retrieve the config for a remote leosac unit.
std::string public_key_
z85 encoded public curve key
CommandHandlerMap command_handlers_
RemoteControlSecurity security_
Object to check remote user permission before processing their request.
bool handle_module_config(zmqpp::message *msg_in, zmqpp::message *msg_out)
Extract and verify content from user-message and call implementation.
void general_config(ConfigManager::ConfigFormat cfg_format, zmqpp::message *msg_out)
Implements GLOBAL_CONFIG API call.
bool handle_module_list(zmqpp::message *msg_in, zmqpp::message *msg_out)
Extract and verify content from message and call implementation.
This class handle the remote control of leosac.