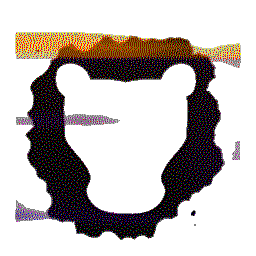 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
26 #include <zmqpp/zmqpp.hpp>
56 std::vector<std::string>
cmd_;
77 const std::string &name,
78 const std::vector<std::string> &auth_contexts,
79 const std::vector<DoormanAction> &actions);
92 const std::vector<std::shared_ptr<DoormanDoor>> &
doors()
const;
107 std::vector<std::shared_ptr<DoormanDoor>>
doors_;
std::vector< DoormanAction > actions_
Leosac::Auth::AccessStatus on_
When should this action be done? on GRANTED or DENIED ?
DoormanInstance & operator=(const DoormanInstance &)=delete
std::string target_
Target component.
Helper struct to wrap an "action".
std::vector< std::shared_ptr< DoormanDoor > > doors_
std::shared_ptr< AuthTarget > AuthTargetPtr
const std::vector< std::shared_ptr< DoormanDoor > > & doors() const
This is the header file for a generated source file, GitSHA1.cpp.
bool ignore_action(const DoormanAction &action, Auth::AccessStatus status) const
Should we ignore this action.
std::vector< std::string > cmd_
The command to be send.
DoormanInstance(DoormanModule &module, zmqpp::context &ctx, const std::string &name, const std::vector< std::string > &auth_contexts, const std::vector< DoormanAction > &actions)
Create a new doorman.
Auth::AuthTargetPtr find_target(const std::string &name) const
Implements a Doorman, that is, a component that will listen to authentication event and react accordi...
std::map< std::string, zmqpp::socket > targets_
Socket (REP) connected to each target this doorman may have.
zmqpp::socket & bus_sub()
void command_send_recv(const std::string &target_name, zmqpp::message msg)
Send a command to a target and wait for response.
void handle_bus_msg()
Activity we care about happened on the bus.
Main class for the module, it create handlers and run them to, well, handle events and send command.