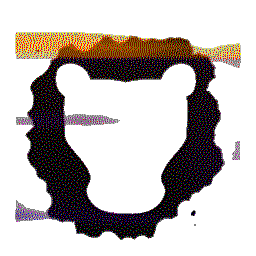 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
39 boost::property_tree::ptree cfg, module_cfg, readers_cfg, reader1_cfg;
41 reader1_cfg.add(
"name",
"WIEGAND_1");
42 reader1_cfg.add(
"high",
"GPIO_HIGH");
43 reader1_cfg.add(
"low",
"GPIO_LOW");
45 readers_cfg.add_child(
"reader", reader1_cfg);
46 module_cfg.add_child(
"readers", readers_cfg);
48 cfg.add(
"name",
"WIEGAND_READER");
49 cfg.add_child(
"module_config", module_cfg);
51 return test_run_module<WiegandReaderModule>(&ctx_, pipe, cfg);
57 , high_(ctx_,
"GPIO_HIGH")
58 , low_(ctx_,
"GPIO_LOW")
60 bus_sub_.subscribe(
"S_WIEGAND_1");
73 for (
int i = 0; i < 32; i++)
78 ASSERT_TRUE(
bus_read(bus_sub_,
"S_WIEGAND_1",
82 for (
int i = 0; i < 32; i++)
91 std::this_thread::sleep_for(std::chrono::milliseconds(2));
94 ASSERT_TRUE(
bus_read(bus_sub_,
"S_WIEGAND_1",
TEST_F(WiegandReaderTest, readCard)
RuntimeOptions class declaration.
This is the header file for a generated source file, GitSHA1.cpp.
virtual bool run_module(zmqpp::socket *pipe) override
Called in the module's actor's thread.
bool bus_read(zmqpp::socket &sub, Content... content)
Make a blocking read on the bus, return true if content match the message.
Unit testing utility class that helps writing test.
Provide support for Wiegand devices.
Base class for test fixtures, it defines a ZMQ context, a BUS and a sub socket connect to the bus.
A test helper class that emulate a GPIO pin.
@ SIMPLE_WIEGAND
This define message formatting for data source SIMPLE_WIEGAND.