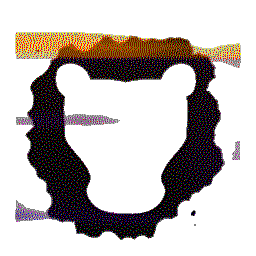 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
38 auto t = std::make_shared<Tasks::GetLocalConfigVersion>(
kernel_);
41 ASSERT_LOG(t->succeed(),
"Tasks::GetLocalConfigVersion failed.");
42 return t->config_version_;
47 boost::property_tree::ptree out;
55 "Retrieving `kernel configuration` from CoreAPI failed.");
62 using namespace std::chrono;
65 auto now = steady_clock::now();
71 ASSERT_LOG(task->succeed(),
"Retrieving `uptime` from CoreAPI failed.");
85 ASSERT_LOG(task->succeed(),
"Retrieving `instance_name` from CoreAPI failed.");
92 std::vector<std::string> out;
99 ASSERT_LOG(task->succeed(),
"Retrieving `modules names` from CoreAPI failed.");
112 ASSERT_LOG(task->succeed(),
"Requesting server restart failed.");
void restart_later()
Set the running_ and want_restart flag so that leosac will restart in the next main loop iteration.
boost::property_tree::ptree kernel_config() const
Retrieve the property tree describing the Leosac's kernel configuration.
#define ASSERT_LOG(cond, msg)
void restart_server() const
Request that Leosac restarts.
This is the header file for a generated source file, GitSHA1.cpp.
const std::chrono::steady_clock::time_point start_time() const
Return the time point at which Leosac started.
static TaskPtr build(const Callable &callable)
uint64_t config_version() const
Retrieve the local configuration version.
uint64_t uptime() const
Returns the uptime, in seconds.
CoreUtilsPtr core_utils()
Returns a (smart) pointer to the core utils: some thread-safe utilities.
const ModuleManager & module_manager() const
Returns a reference to the module manager object (const version).
std::string instance_name() const
Returns the instance_name of Leosac.
ConfigManager & config_manager()
Retrieve a reference to the configuration manager object.
std::vector< std::string > modules_names() const
Retrieve the names of all enabled modules.