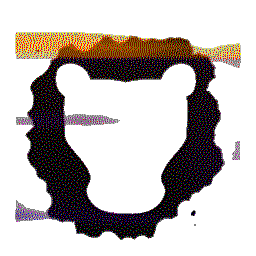 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
30 #include <boost/property_tree/ptree_fwd.hpp>
41 _enabled = cfg.get<
bool>(
"enabled",
false);
45 _interface = cfg.get<std::string>(
"interface");
47 _netmask = cfg.get<std::string>(
"netmask");
48 _defaultIp = cfg.get<std::string>(
"default_ip");
50 _gateway = cfg.get<std::string>(
"gateway");
52 INFO(
"Network settings:" << std::endl
53 <<
'\t' <<
"enabled=" <<
_enabled << std::endl
55 <<
'\t' <<
"ip=" <<
_ip << std::endl
56 <<
'\t' <<
"netmask=" <<
_netmask << std::endl
57 <<
'\t' <<
"gateway=" <<
_gateway << std::endl
61 INFO(
"Network configuration disabled. Will not touch system setting.");
80 INFO(
"JUST LOADED IFCONFIG CONFIGURATION");
92 INFO(
"DHCP set to" << enabled);
100 INFO(
"CustomIP set to" << enabled);
UnixShellScript class declaration.
void setDHCP(bool enabled)
void setEnabled(bool state)
This is the header file for a generated source file, GitSHA1.cpp.
NetworkConfig class declaration.
static constexpr const char * NetCfgFile
void setCustomIP(bool enabled)
NetworkConfig(const Kernel &k, const boost::property_tree::ptree &cfg)
std::string script_directory() const
Return the path to the scripts directory.