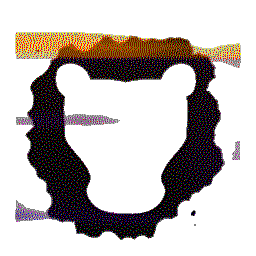 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
42 virtual bool isAccessGranted(
const std::chrono::system_clock::time_point &date,
56 virtual const std::map<std::string, std::vector<Tools::IScheduleCPtr>> &
68 std::map<std::string, std::vector<Tools::IScheduleCPtr>>
schedules_;
std::map< std::string, std::vector< Tools::IScheduleCPtr > > schedules_
Map target name to target's schedules.
virtual const std::vector< Tools::IScheduleCPtr > & defaultSchedules() const
std::vector< Tools::IScheduleCPtr > default_schedule_
Schedule for default target.
std::shared_ptr< AuthTarget > AuthTargetPtr
virtual void addAccessSchedule(AuthTargetPtr target, const Tools::IScheduleCPtr &sched)
Adds a schedule where access to a given target is allowed.
This is the header file for a generated source file, GitSHA1.cpp.
Concrete implementation of a simple access control class.
virtual const std::map< std::string, std::vector< Tools::IScheduleCPtr > > & schedules() const
Returns the map of schedule for each target (except the default target)
virtual size_t schedule_count() const override
Returns the number of schedule associated with the profile.
virtual bool isAccessGranted(const std::chrono::system_clock::time_point &date, AuthTargetPtr target) override
Does the profile allow access to the user.
Holds information about access permission.