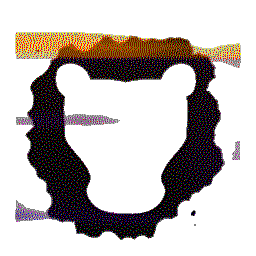 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include <boost/date_time/posix_time/posix_time.hpp>
24 #include <odb/core.hxx>
39 #pragma db object optimistic
94 #pragma db view object(LogEntry)
97 #pragma db column("count(" + LogEntry::id_ + ")")
static QueryResult retrieve(DBPtr database, int page_number, int page_size, bool order_asc)
std::vector< LogEntry > entries
std::shared_ptr< odb::database > DBPtr
This is the header file for a generated source file, GitSHA1.cpp.
Structure holding the result for a retrieve() call.
boost::posix_time::ptime timestamp_
std::string run_id_
The run_id is generated when Leosac starts, and it is used to identify "runs" (ie if Leosac restarts)...