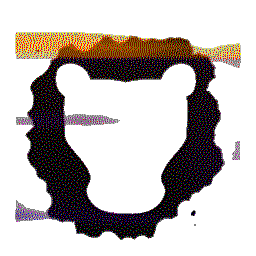 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include <condition_variable>
76 template <
typename Callback>
88 template <
typename Callback>
100 template <
typename Callback>
106 const std::string &
get_guid()
const;
109 virtual bool do_run() = 0;
120 std::condition_variable
cv_;
std::function< void(void)> on_completion_
void set_on_failure(Callback c)
Set a callback that will be invoked if and when the task fails.
A base class for a tasks.
std::function< void(void)> on_success_
This is the header file for a generated source file, GitSHA1.cpp.
std::condition_variable cv_
Task & operator=(const Task &)=delete
void set_on_completion(Callback c)
Set a callback that will be invoked when the tasks is completed, no matter if it succeeded or not.
void wait()
Instead of spinlocking over is_complete() one can call wait() to hum...
std::function< void(void)> on_failure_
std::atomic_bool complete_
bool is_complete() const
Has the tasks completed its execution.
void set_on_success(Callback c)
Set a callback that will be invoked if and when the task succeed.
const std::string & get_guid() const
std::exception_ptr get_exception() const