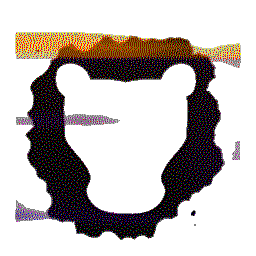 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
23 #include <zmqpp/zmqpp.hpp>
72 const std::string &
name()
const;
FExternalServer & operator=(const FExternalServer &)=delete
zmqpp::socket backend_
A socket to talk to the backend server.
bool connect()
Connect to the server.
Facade object for a Wiegand Reader device.
This is the header file for a generated source file, GitSHA1.cpp.
bool disconnect()
Disconnect from the server.
~FExternalServer()=default
FExternalServer(zmqpp::context &ctx, const std::string &server_name)
Construct a facade to an external server; this facade will connect to the server.
bool isConnected() const
Check if is connected to the server.
const std::string & name() const
Returns the device's name.
bool send_to_backend(zmqpp::message &m)
Send a message to the backend_ server and wait for a response.