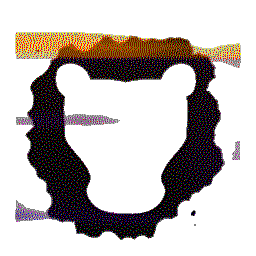 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
33 using TimePoint = std::chrono::system_clock::time_point;
virtual const TimePoint & generated_at() const =0
std::shared_ptr< AuditEntry > AuditEntryPtr
unsigned long AuditEntryId
This is the header file for a generated source file, GitSHA1.cpp.
virtual ~IUpdate()=default
virtual Audit::AuditEntryId get_checkpoint() const =0
std::chrono::system_clock::time_point TimePoint
virtual const std::string & description() const =0
virtual const TimePoint & status_updated_at() const =0
virtual Status status() const =0
virtual const std::string & source_module() const =0
virtual void set_checkpoint(Audit::AuditEntryPtr)=0
Set the checkpoint for the update object.
virtual UpdateId id() const =0