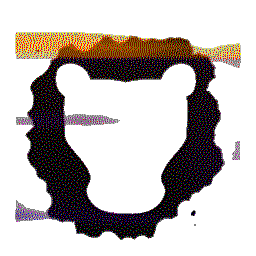 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
24 #include <libscrypt.h>
27 #include <boost/algorithm/hex.hpp>
28 #include <boost/algorithm/string/classification.hpp>
29 #include <boost/algorithm/string/split.hpp>
70 template <
typename SerializedType>
77 static std::string
Hex(
const std::vector<uint8_t> &in)
80 boost::algorithm::hex(in.begin(), in.end(), back_inserter(res));
84 static std::vector<uint8_t>
UnHex(
const std::string &in)
86 std::vector<uint8_t> res;
87 boost::algorithm::unhex(in.begin(), in.end(), back_inserter(res));
93 return std::vector<uint8_t>(in.begin(), in.end());
102 std::stringstream ss;
115 std::vector<std::string> bla;
116 boost::algorithm::split(bla, in, boost::algorithm::is_any_of(
":"));
119 throw std::runtime_error(
"Cannot unserialize.");
121 result.
p.
N = std::stoi(bla[0]);
122 result.
p.
r = std::stoi(bla[1]);
123 result.
p.
p = std::stoi(bla[2]);
144 const std::vector<uint8_t> &salt,
static std::vector< uint8_t > UnHex(const std::string &in)
static bool Verify(const std::vector< uint8_t > &in, const ScryptResult &expected)
Verify that the input in, when hashed, correspond to the expected ScryptResult.
Interface to serialize a ScryptResult to a SerializedType object.
bool operator==(const ScryptResult &o) const
static std::string Hex(const std::vector< uint8_t > &in)
virtual ScryptResult UnSerialize(const SerializedType &in) const =0
bool operator==(const ScryptParam &o) const
virtual ScryptResult UnSerialize(const std::string &in) const override
std::vector< uint8_t > hash
The hash.
std::vector< uint8_t > salt
Salt used for generation.
bool operator!=(const ScryptResult &o) const
virtual std::string Serialize(const ScryptResult &in) const override
static ScryptParam default_
virtual SerializedType Serialize(const ScryptResult &in) const =0
static std::vector< uint8_t > ToByteVector(const std::string &in)
static ScryptResult Hash(const std::vector< uint8_t > &in, const std::vector< uint8_t > &salt, const ScryptParam ¶m=default_)
Wrapper around low-level hash function.
ScryptParam p
Parameters used to generated the hash.