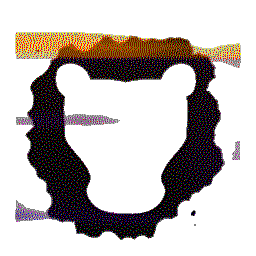 |
Leosac
0.8.0
Open Source Access Control
|
Go to the documentation of this file.
21 #include "gtest/gtest.h"
30 TEST(VersionTest, buildVersionString)
36 TEST(VersionTest, versionCompare)
44 TEST(VersionTest, isVersionValid)
This is the header file for a generated source file, GitSHA1.cpp.
TEST(VersionTest, isVersionValid)